Design patterns are the backbone of good software architecture. They are tried and tested solutions to common problems or scenarios that arise in software design.
They provide standard terminology and are a general repeatable solution to a commonly occurring problem. In essence, they are templates used to solve problems that can be used in many different situations. This article delves deeper into the basics if you’re interested.
Table of Contents
- Design Patterns’ Importance
- Ideal Moments for Patterns
- Implementing Patterns
- JavaScript Pattern Examples
- Resources
- Knowledge Quiz
Why Are Design Patterns Essential?
1. Reusability
Design patterns make it possible to use code more than once. Developers can use them as a form of template to solve a specific issue, which can subsequently be applied to other areas of the program or even new projects. This reduces the need to reinvent the wheel, resulting in faster development.
2. Improved Communication
Design patterns have a universal language of their own, which enhances communication among developers. When a developer mentions the name of the design pattern, it conveys a lot more meaning, enabling efficient and swift conversations around complex design scenarios.
3. Reduced Complexity
Software design complexity can be reduced with the use of design patterns. Providing a ready-made architecture for solving a particular problem simplifies the design process and makes the code more organized.
4. Enhanced Code Quality
The use of design patterns results in better code quality. They promote code reuse and encapsulation, ensuring that the system is modular and that changes in one part of the system do not impact others.
Mastering design patterns in software development is like learning the keys on a piano; it empowers you to create a beautiful symphony in your code, harmonizing complexity with efficiency and scalability.
Identifying the Right Moment for Design Patterns
1. At the Start of a New Project
Using design patterns to structure your application architecture might be advantageous when starting a new project. It provides developers with a clear roadmap and simplifies the coding and debugging process.
2. While Refactoring Existing Code
Often, during the refactoring process, one may encounter code that could be easier to work with. In such situations, applying the relevant design patterns can be a game-changer. They help simplify the code and make it easier to understand and modify.
3. Adding New Functionality to Existing Code
Adding new features to existing code can sometimes be challenging, especially if the existing code is complex or not well-organized. Here, design patterns can help by giving an organized way to add new features without causing too much trouble.
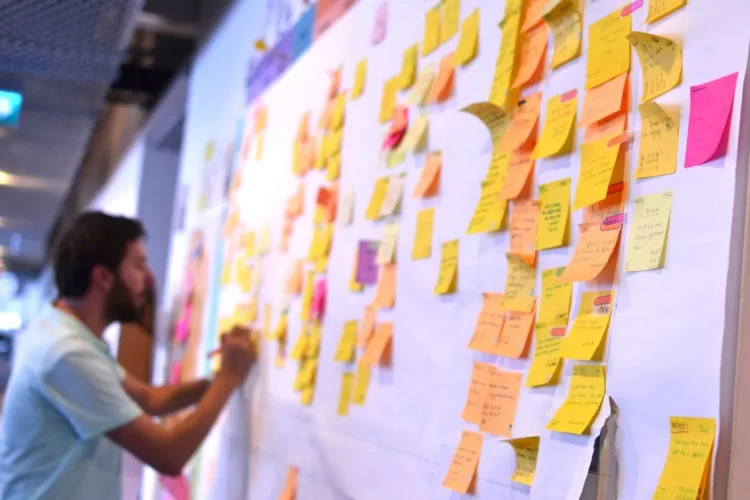
Mastering the Implementation of Design Patterns
Understanding and applying design patterns requires a systematic approach.
1. Understanding the Pattern
Start by thoroughly understanding the design pattern’s structure, intent, and application. Numerous resources are available, including the classic Design Patterns: Elements of Reusable Object-Oriented Software by the Gang of Four (GoF), which you may find beneficial.
2. Identifying the Applicability
Once you understand the pattern, look for places in your program to use it. Applying them unnecessarily might lead to additional complexity.
3. Adapting and Implementing the Pattern
After identifying where to apply, adapt the pattern as per your specific requirements and implement it in your code. You must always consider your specific project requirements and constraints when adapting a pattern.
Exploring Design Patterns with JavaScript: Practical Examples
Understanding when and how to use design patterns is key to maximizing their benefits. Let’s explore how to apply some of the most common design patterns with examples.
1. Creational Patterns: Singleton
Creational patterns deal with object-creation mechanisms. In many cases, we must ensure that a class has just one instance and that there is a global point of access to it.
For instance, think about a logging class. This class could be used to log errors and exceptions, access logs, etc. Using a Singleton pattern ensures that all parts of the app use a single log file.
let instance = null;
class LoggerSingleton {
constructor(filePath) {
if (!instance) {
instance = this;
instance.filePath = filePath;
}
return instance;
}
log(data) {
// Here, we'll just print data to console
console.log(`Logging data: ${data}`);
}
}
const logger1 = new LoggerSingleton('/path/to/mylogfile.log');
const logger2 = new LoggerSingleton('/path/to/differentlogfile.log');
logger1.log('This is a log entry'); // 'Logging data: This is a log entry'
logger2.log('This is another log entry'); // 'Logging data: This is another log entry'
2. Structural Patterns: Adapter
Structural patterns are concerned with the composition of classes and objects into larger structures. For instance, the Adapter pattern acts as a bridge between two incompatible interfaces. This pattern combines the capability of two independent interfaces.
Imagine you’re integrating a legacy system with a new system, and their interfaces are incompatible. Here, an adapter class can be used to bridge the gap.
Consider we have a legacy system that uses XML and a new system that uses JSON. These two systems need to communicate, but their interfaces are incompatible. Here, an adapter can be used to convert XML data to JSON and vice versa.
class XMLSystem {
constructor() {
this.getXMLData = () => {
// fetches XML data
let data = 'Hello World!';
return data;
};
}
}
class JSONSystem {
constructor() {
this.getJSONData = () => {
// fetches JSON data
let data = { message: 'Hello World!' };
return data;
};
}
}
class Adapter {
constructor() {
this.jsonSystem = new JSONSystem();
this.getXMLData = () => {
let jsonData = this.jsonSystem.getJSONData();
// convert JSON data to XML
let xmlData = '' + jsonData.message + '';
return xmlData;
};
}
}
let adapter = new Adapter();
console.log(adapter.getXMLData()); // 'Hello World!'
3. Behavioral Patterns: Observer
Behavioral patterns are concerned with the interaction and responsibility of objects. A perfect example is the Observer pattern, where an object (known as a subject) maintains a list of objects depending on it (observers), automatically notifying them of any state changes.
An example of this could be a newsletter system, where subscribers are notified whenever a new article is published.
class Publisher {
constructor() {
this.subscribers = [];
}
subscribe(subscriber) {
this.subscribers.push(subscriber);
}
notify(data) {
this.subscribers.forEach(subscriber => subscriber.receive(data));
}
}
class Subscriber {
receive(data) {
console.log(`New article published: ${data}`);
}
}
const publisher = new Publisher();
const subscriber1 = new Subscriber();
const subscriber2 = new Subscriber();
publisher.subscribe(subscriber1);
publisher.subscribe(subscriber2);
publisher.notify('Understanding Design Patterns');
// Logs 'New article published: Understanding Design Patterns' on all subscribers
Remember, these examples are simple and are meant to give a high-level idea of how these patterns work.
Each of these patterns can be explored in much more depth, with more complex examples and variations to meet specific requirements.
By understanding and using design patterns effectively, we can improve the efficiency of our software development process and produce code that is more maintainable, scalable, and robust. As with any tool or method, knowing when and how to use it right is important.
Resources
For further reading and to deepen your understanding of Design Patterns, you might find these resources useful:
- SourceMaking: Design Patterns
- Book: Design Patterns: Elements of Reusable Object-Oriented Software
- Refactoring Guru: Design Patterns
- TutorialsPoint: Design Pattern Tutorial
- DoFactory: .NET Design Patterns
Test Your Knowledge: Design Patterns Quiz
Here’s a little quiz to test your understanding of the concepts covered in this blog post. Feel free to leave your answers in the comment section. We would love to see your responses!
1. What are Design Patterns?
- Templates used to solve problems.
- Coding guidelines.
- Programming languages.
2. When can Design Patterns be useful? (You can choose more than one option)
- When starting a new project.
- While refactoring existing code.
- When debugging a program.
3. What is an example of a creational design pattern?
4. How does the Observer pattern work?
Remember, there are no right or wrong answers. It’s all about learning and growing together. So, don’t hesitate to share your thoughts below!
Conclusion
Design patterns are powerful tools for software design. They offer tried-and-true solutions to common coding problems, facilitate clear and efficient communication among developers, and significantly enhance the quality of the code.
But they are not magic solutions and should be used carefully, considering the project’s needs and limits.
Remember that the goal is to fix problems quickly and effectively and that design patterns are just one way to do that.
Learning and applying the design patterns of your project will help you become a competent developer.
🚀 Before You Go:
- 👏 Found this guide helpful? Give it a like!
- 💬 Got thoughts? Share your insights!
- 🔄 Know someone who needs this? Share the post!
🌟 Your support keeps us going!
📬 Want more like this? Get updates straight to your inbox!
Related Posts
Don’t Be Scared, Devin AI + You = Super Developer
Explore the transformative power of Devin AI in the software development industry. Learn how this autonomous AI software engineer can...
20 Things You Should Consider When You Grow as a Developer
Explore 20 key strategies designed to propel developers at any stage of their career toward greater success. Learn how to...
Security Debt in Software Engineering Comprehensive Overview
Explore the critical concept of security debt in software development, including its impact, management strategies, and the best tools for...